In our last example of working with dictionaries, we showed you How To Delete a Set of Keys From a Python Dictionary. This is a very useful scenario if you want to tidy up the dictionary and remove data not required.
Following on from that, in this post, we are going to look at changing key names. You may find that you have the dictionary you want but you need to change some of the key names to ensure the proper names are consistent with other parts of your program.
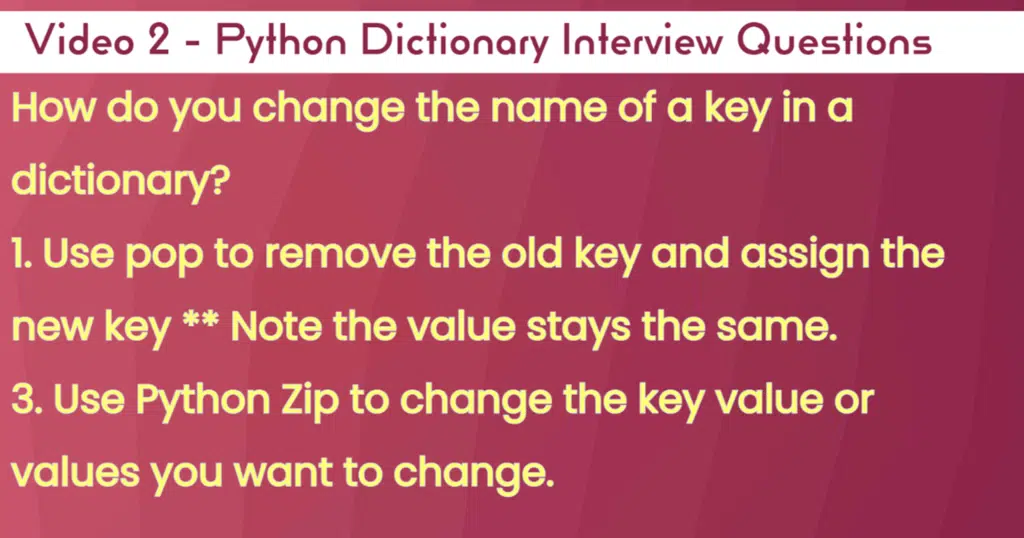
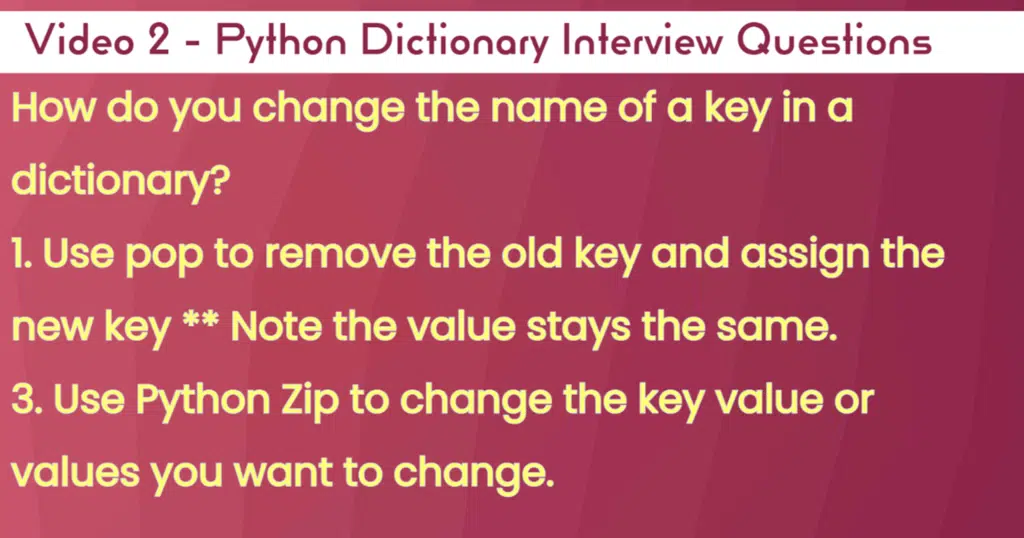
Option1 uses the pop method :
- Create a dictionary with the key-value pairs in it. Note that this will also be used for the second option.
- Then this line European_countries[“United Kingdom”] = European_countries.pop(“UK”) basically says to find “UK” in the values, drop it and replace it with the value “United Kingdom”
Before the values using the pop method where:
{‘Ireland’: ‘Dublin’, ‘France’: ‘Paris’, ‘UK’: ‘London’}
After the values using the pop method are:
{‘Ireland’: ‘Dublin’, ‘France’: ‘Paris’, ‘United Kingdom’: ‘London’}
Option 2 uses the zip method :
- In this scenario, we have created a dictionary called European countries.
- the objective here is to replace the names with the three-letter country code.
- Note it updates the values into new_dict using zip, and using the update_elements list, based on the order they are in, in both.
Before the values using the zip method where:
{‘Ireland’: ‘Dublin’, ‘France’: ‘Paris’, ‘United Kingdom’: ‘London’}
After the values using the zip method are:
{‘IRE’: ‘Dublin’, ‘FR’: ‘Paris’, ‘UK’: ‘London’}
#Slide 9
# How do you change the name of a key in a dictionary
#1. Create a new key , remove the old key, but keep the old key value
# create a dictionary
European_countries = {
"Ireland": "Dublin",
"France": "Paris",
"UK": "London"
}
print(European_countries)
#1. rename key in dictionary
European_countries["United Kingdom"] = European_countries.pop("UK")
# display the dictionary
print(European_countries)
#2. Use zip to change the values
European_countries = {
"Ireland": "Dublin",
"France": "Paris",
"United Kingdom": "London"
}
update_elements=['IRE','FR','UK']
new_dict=dict(zip(update_elements,list(European_countries.values())))
print(new_dict)
We hope you enjoyed this, we have plenty of videos that you can look at to improve your knowledge of Python here: Data Analytics Ireland Youtube